MOSTLY AI Python client
A Python wrapper for the MOSTLY AI platform.
Intent | Primitive |
---|---|
Train a Generative AI on tabular data | g = mostly.train(data) |
Empower your team with safe synthetic data | mostly.share(g, email) |
Generate unlimited synthetic data on demand | mostly.generate(g, size) |
Prompt the generator for the data that you need | mostly.generate(g, seed) |
Connect to any data source within your org | mostly.connect(config) |
Check your daily usage and credits | mostly.me() |
Installation
bash
pip install mostlyai
Get an API key
To use the Python client, you need an API key. Get your API key for the REST API or Python client from your user profile menu.
Steps
- Hover over the profile menu in the upper right and select API key.
- Click Generate API Key.
What's next
Your key is immediately copied to your clipboard. You can now use it for the REST API or to instantiate your Python client.
python
from mostlyai import MostlyAI
mostly = MostlyAI(api_key="mostly-**********")
Examples
As you explore the Generators, Synthetic datasets, and Connectors pages, you will find code snippets that show how to accomplish a task with the Python client. Use the Python and UI tabs to switch between the code snippets and the UI steps.
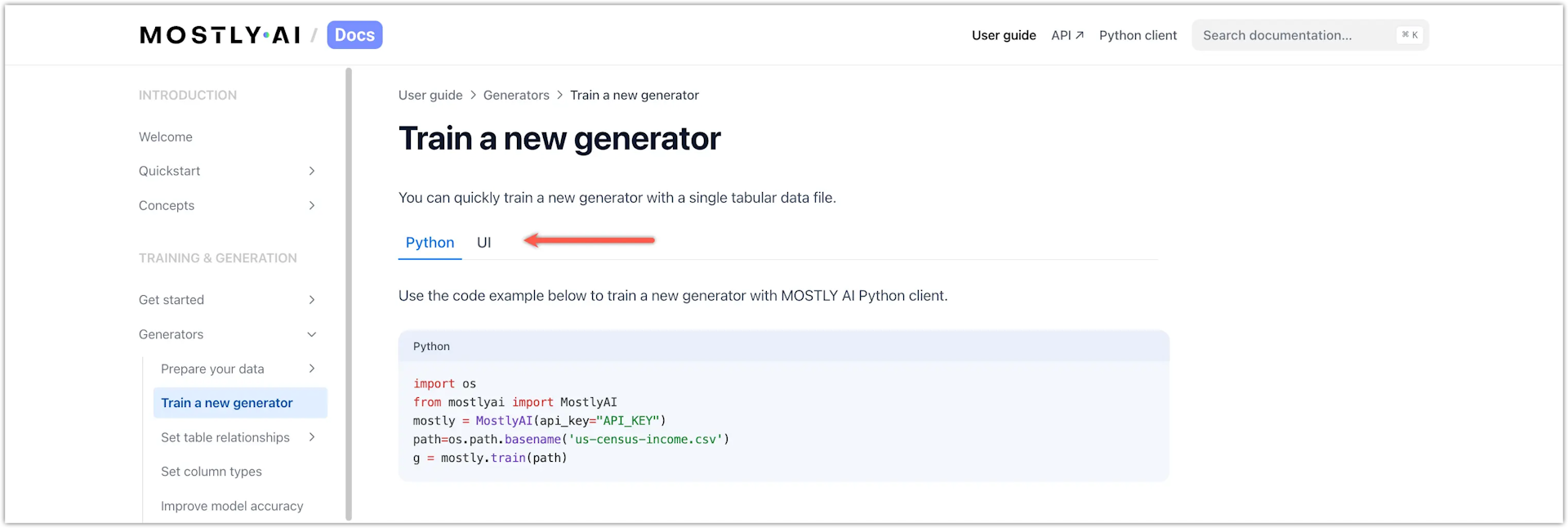
Basic Usage
python
from mostlyai import MostlyAI
mostly = MostlyAI(api_key='your_api_key')
g = mostly.train(data) # train a generator on your data (Pandas DataFrame, URL to CSV, or a connector location)
sd = mostly.generate(g) # generate a synthetic dataset
syn = sd.data() # consume synthetic as pd.DataFrame
Supported Methods
Connectors
python
c = mostly.connect(config)
c = mostly.connectors.create(config)
c = mostly.connectors.get(id)
it = mostly.connectors.list()
c = c.update(config)
ls = c.locations(prefix)
config = c.config()
c.delete()
Generators
python
g = mostly.train(data, config, name, start=True, wait=True)
g = mostly.generators.create(config)
g = mostly.generators.get(id)
it = mostly.generators.list()
g = g.update(config)
config = g.config()
g.delete()
g.training.start()
g.training.progress()
g.training.cancel()
g.training.wait()
Synthetic Datasets
python
sd = mostly.generate(g, seed=seed)
sd = mostly.generate(g, size=size)
sd = mostly.generate(g, config=config)
sd = mostly.synthetic_datasets.create(g, config)
sd = mostly.synthetic_datasets.get(id)
it = mostly.synthetic_datasets.list()
config = sd.config()
sd.delete()
sd.generation.start()
sd.generation.progress()
sd.generation.cancel()
sd.generation.wait()
sd.data()
sd.download(file, format)
Synthetic probes
python
sp = mostly.probe(g, seed=seed)
sp = mostly.probe(g, size=size)
sp = mostly.probe(g, config=config)
Sharing
python
mostly.share(g | sd | c, email)
mostly.unshare(g | sd | c, email)
g.shares()
sd.shares()
c.shares()
Usage
python
mostly.me()